Cellular Automata
I am currently reading Stephen Wolfram's A New Kind of Science
In Chapter 3, he discusses cellular automata. I thought it would be fun to try to implement a few of them.
The state of a cell is determined by the state of it and it's neighbors in the previous row. There are 8 possible combinations of three cells, so 256 possible sets of cellular automata rules. Wolfram's numbering system assigns a 0 or 1 to each configuration if the next cell will be white or black. The resulting 8 digit binary number is converted to base 10.
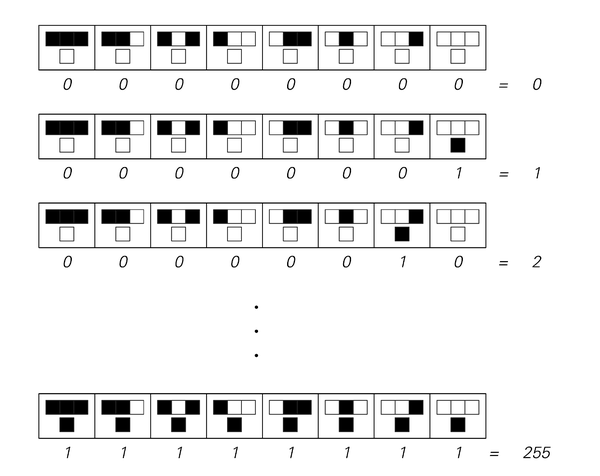
Wolfram's numbering system
Try it:
Enter a number between 0 and 255 to see the corresponding cellular automata.
Note: these start with a single black cell in the middle of the first row unless randomize is selected. Also the left and right edges are connected.
Rule 45
Rule 30 (00011110) - A cell is set to the color of it's left neighbor in the previous row if it and it's right neighbor are white in the previous row. Otherwise it is set to the opposite value of that left neighbor.
if (previousRight === 0 && previousCenter === 0) {
grid[col][row] = previousLeft;
} else {
grid[col][row] = previousLeft === 0 ? 1 : 0;
}
Rule 90 (01011010) - A cell is black if either (but not both) of it's neighbors were black on the previous row.
if (previousLeft ^ previousRight) {
grid[col][row] = 1;
}
Rule 254 (11111110) - A cell is black if it or it's left or right neighbor were black in the previous row.
if (previousLeft || previousRight || previousCenter) {
grid[col][row] = 1;
}